감성 자동제어
[안드로이드 스튜디오] try-catch문에 대해 알아보자!
안녕하세요!! 안드로이드 스튜디오 예외처리에 대해서 알아보도록 하겠습니다! 계산기를 예시로 알아볼도록 할텐데! 아마도 계산기 예제의 문제를 만들고 난 뒤 소수점을 입력하거나 정수가 아닌 수 또는 텍스트가 입력될 때 앱이 강제로 종료되는 현상을 겪은 분이 있다면 이 방법을 사용해서 문제를 해결해보도록 하세요! 그럼 시작하겠습니다!!
설명
1) "Edit text" 에 2개의 숫자를 입력받아 두 숫자를 더하도록 하겠습니다.
2) 예외처리를 한 버튼, 예외처리를 하지 않은 버튼 2개의 버튼을 만듭니다.
3) 더한값을 "Textview"에 나타내도록 하겠습니다.
4) 만약 예외처리를 실행했을 경우 토스트 메시지를 이용하여 표현해주도록 하겠습니다.
Activity_main.xml(layout)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
|
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_margin="5dp"
android:orientation="vertical"
tools:context=".MainActivity">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<EditText
android:id="@+id/edit_text1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:hint="숫자1"
android:textSize="20dp" />
<EditText
android:id="@+id/edit_text2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:hint="숫자2"
android:textSize="20dp" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<Button
android:id="@+id/add_error_button"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="더하기(예외처리x)"
android:textSize="25dp" />
<Button
android:id="@+id/add_nomal_button"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="더하기(예외처리o)"
android:textSize="25dp" />
</LinearLayout>
<TextView
android:id="@+id/result"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="결과 값 : "
android:textSize="30dp" />
</LinearLayout>
|
cs |
MainActivity(Class)
1) try문 안에는 "예외처리 예상 코드" 를 작성해줍니다.
-> 저의경우 정수값을 이용해서 더하기를 해줘야 하는데 정수값이 아닌경우 예외가 일어나게 됩니다.
2) catch문 안에는 예외 발생시 처리할 코드"를 작성해줍니다.
-> 저는 토스트메시지를 이용하였습니다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
|
package com.example.try_catch;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
EditText editText1, editText2;
Button error_button, nomal_button;
TextView textView_result;
String num1, num2;
Integer result;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
editText1 = findViewById(R.id.edit_text1);
editText2 = findViewById(R.id.edit_text2);
error_button = findViewById(R.id.add_error_button);
nomal_button = findViewById(R.id.add_nomal_button);
textView_result = findViewById(R.id.result);
//예외처리가 아닌 버튼을 눌렀을 때
error_button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
num1 = editText1.getText().toString();
num2 = editText2.getText().toString();
result = Integer.parseInt(num1) + Integer.parseInt(num2);
textView_result.setText("결과 값 : " + result);
}
});
//예외처리 버튼을 눌렀을 때
nomal_button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
try {
//예외 발생 예상 코드
num1 = editText1.getText().toString();
num2 = editText2.getText().toString();
result = Integer.parseInt(num1) + Integer.parseInt(num2);
textView_result.setText("결과 값 : " + result);
} catch (Exception e) {
//예외 발생시 처리할 내용
Toast.makeText(MainActivity.this, "정수를 입력해 주세요.", Toast.LENGTH_SHORT).show();
}
}
});
}
}
|
cs |
결과화면
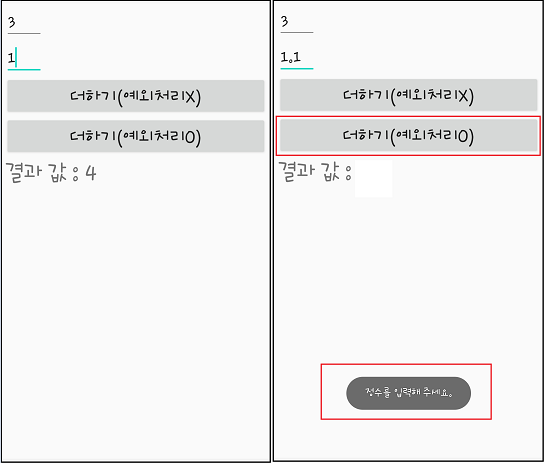
마무리
이상으로 try-catch문 예외처리에 대해 알아보았습니다! 긴 글 읽어주셔서 감사합니다!!
'안드로이드 스튜디오' 카테고리의 다른 글
[안드로이드 스튜디오] 난수(Random) 숫자 발생시키는 방법! (0) | 2020.12.06 |
---|---|
[안드로이드 스튜디오] 기초 완전 간단한 계산기 앱 만들기! (0) | 2020.11.30 |
[안드로이드 스튜디오] XML을 이용한 옵션메뉴 만들기! (0) | 2020.11.25 |
[안드로이드 스튜디오] 대화상자(dialog) 사용하는 방법-AlertDialog (0) | 2020.11.23 |
[안드로이드 스튜디오] 버튼 클릭시 이미지 변경!! (4) | 2020.11.20 |