감성 자동제어
[안드로이드 스튜디오] 간단한 계산기 앱 만들기!!
안녕하세요 간단한 계산기 앱을 만드는 방법에 대해 알아보도록 하겠습니다! 먼저 구성에 대해 알아보도록 하겠습니다.
EditText 2개 = 값을 계산 할 숫자를 입력받습니다.
Button 4개 = 더하기, 빼기, 곱하기, 나누기 버튼입니다.
TextView 1개 = 결과값을 띄워서 확인합니다.
Activity_main.xml(layout)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
|
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/layout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_margin="10dp"
android:orientation="vertical"
tools:context=".MainActivity">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="0.2"
android:gravity="center"
android:orientation="vertical">
<EditText
android:id="@+id/Edit_1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:hint="첫 번째 숫자"
android:textSize="25dp" />
<EditText
android:id="@+id/Edit_2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:hint="두 번째 숫자"
android:textSize="25dp" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="1"
android:orientation="vertical">
<Button
android:id="@+id/btn_add"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="더하기"
android:textSize="25dp" />
<Button
android:id="@+id/btn_sub"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="빼기"
android:textSize="25dp" />
<Button
android:id="@+id/btn_mul"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="곱하기"
android:textSize="25dp" />
<Button
android:id="@+id/btn_div"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="나누기"
android:textSize="25dp" />
</LinearLayout>
<TextView
android:id="@+id/Text_result"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="계산결과 : "
android:textColor="#171717"
android:textSize="40dp" />
</LinearLayout>
|
cs |
MainActivity(Class)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
|
public class MainActivity extends AppCompatActivity {
EditText edit1, edit2;
Button btn_add, btn_sub, btn_mul, btn_div;
TextView text_Result;
String num1, num2;
Integer result;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
edit1 = findViewById(R.id.Edit_1);
edit2 = findViewById(R.id.Edit_2);
btn_add = findViewById(R.id.Btn_add);
btn_sub = findViewById(R.id.Btn_sub);
btn_mul = findViewById(R.id.Btn_mul);
btn_div = findViewById(R.id.Btn_div);
text_Result = findViewById(R.id.Text_result);
btn_add.setOnTouchListener(new View.OnTouchListener() {
@Override
public boolean onTouch(View v, MotionEvent event) {
num1 = edit1.getText().toString();
num2 = edit2.getText().toString();
result = Integer.parseInt(num1) + Integer.parseInt(num2);
text_Result.setText("계산결과 : " + result.toString());
return false;
}
});
btn_sub.setOnTouchListener(new View.OnTouchListener() {
@Override
public boolean onTouch(View v, MotionEvent event) {
num1 = edit1.getText().toString();
num2 = edit2.getText().toString();
result = Integer.parseInt(num1) - Integer.parseInt(num2);
text_Result.setText("계산결과 : " + result.toString());
return false;
}
});
btn_mul.setOnTouchListener(new View.OnTouchListener() {
@Override
public boolean onTouch(View v, MotionEvent event) {
num1 = edit1.getText().toString();
num2 = edit2.getText().toString();
result = Integer.parseInt(num1) * Integer.parseInt(num2);
text_Result.setText("계산결과 : " + result.toString());
return false;
}
});
btn_div.setOnTouchListener(new View.OnTouchListener() {
@Override
public boolean onTouch(View v, MotionEvent event) {
num1 = edit1.getText().toString();
num2 = edit2.getText().toString();
result = Integer.parseInt(num1) / Integer.parseInt(num2);
text_Result.setText("계산결과 : " + result.toString());
return false;
}
});
}
}
|
cs |
결과화면
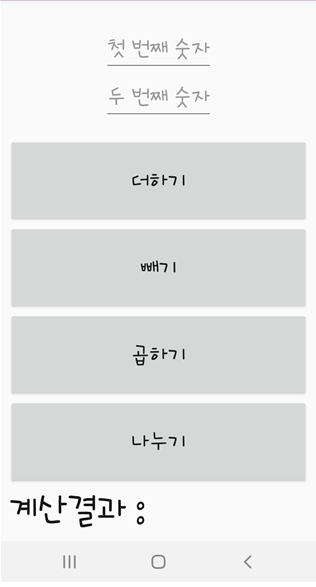
마무리
이상으로 간단한 계산기를 만들어 보았습니다. 아마 소수점을 입력하거나 텍스트를 입력하고 버튼을
누르면 오류가 나며 앱이 종료가 되는 현상이 나타납니다. 이번글에는 간단한 사칙연산을 하기위한 방법에 대해 먼저 알아보기 위해 최대한 간단하게 작성하였습니다. 다음글에는 이것을 보완하여 업그레이드된 계산기를 만들어 보도록 하겠습니다. 감사합니다!!
'안드로이드 스튜디오' 카테고리의 다른 글
[안드로이드 스튜디오] 예외처리 try - catch문 사용방법!(계산기) (0) | 2020.12.22 |
---|---|
[안드로이드 스튜디오] 난수(Random) 숫자 발생시키는 방법! (0) | 2020.12.06 |
[안드로이드 스튜디오] XML을 이용한 옵션메뉴 만들기! (0) | 2020.11.25 |
[안드로이드 스튜디오] 대화상자(dialog) 사용하는 방법-AlertDialog (0) | 2020.11.23 |
[안드로이드 스튜디오] 버튼 클릭시 이미지 변경!! (4) | 2020.11.20 |